CARD HOLDER NAME HERE
MM / YY
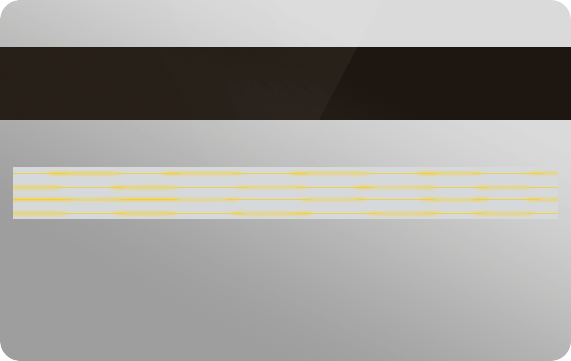
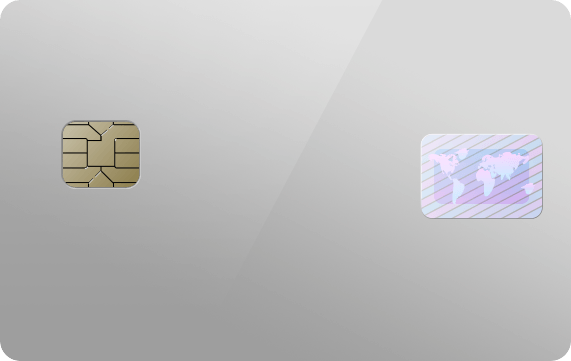
Let's Use Javascript to Detect and Show which Credit Card is being entered. We're also going to use some CSS to "flip" the card over and reveal the back like we're in real life!
OUR PROBLEM
You're given the challenge of creating a new checkout form for your company. The credit card form is not performing well, so you need to make it perform better. What should your first step be? Change the submit button color and run an A/B test on that! You could also try out different headlines or use a sick graphic of someone entering their real CREDIT cards into payment processing systems - this will give users some sense trust when finalizing their purchase at checkout while adding new digital elements onto our pages which are sure worth exploring in more detail if we want our company's conversion rates to increase even further
Why You might need this
- Use Javascript to detect the correct type of credit card
- Simulate a real credit card
- We can do this with a combination of Javascript & jQuery
- This should be cross browser compatible and secure
ANSWER: Let's Use jQuery and CSS
Adding this to any modern checkout page will add extra authenticity in their experience. Why not use an innovative CSS transition that simulates entering your credit card information in person!
Let's start with the pseudo code here. First, we'll want to create the HTML form and a placeholder graphic to represent our credit card. Next we'll need to add a placeholder image for the back of the card that will "reveal" on focus. For the placeholder graphic we use a blank credit card. We use CSS to position the text where it should show up in a real life card. For the javascript we'll need to create 1 function to format all the form data and display it on the pretend credit card. We'll set up our variables like a c++ program.
You'll need to "GET" the card number value and use the jQuery .match function to check if the card is VISA or Mastercard. I use the keyup method to trigger our function everytime someone types in the form. We use .blur and .change methods on the other form inputs to format and trigger the display on the pretend card. The CSS below handles all the flips and z-indexing/ element positioning.
View Code Example Below:
PSEUDO CODE
//Let's define our array of images
//Let's preload our images to the DOM
//** Very important to have these files preloaded before we run our script
//at the end of the preload loop let's run our function (this way we know the files were loaded first)
//Write a function that changes the src of the image every 2 seconds (length of time we want the image to show for)
HTML
<div id="mycard" class="">
<div class="mycard_inner">
<div class="card_content">
<p class="cardvalue">
</p>
<p class="p_cvv float-right" style="visibility: hidden;">
<small class="cvvval">
</small>
</p>
<p class="cardholdername" style="visibility: visible;">
<span class="fname">
CARD HOLDER</span>
<span class="lname">
NAME HERE</span>
</p>
<p class="exp float-left" style="visibility: visible;">
<br>
<span class="expm">
MM</span>
/<span class="expyr">
YY</span>
</p>
<div class="clearfix">
</div>
</div>
</div>
<div class="flipper">
<!-- container holds the back of the card-->
<div class="mycard_back">
<img class="img-fluid center-block as_gen_back card_size" src="img/cvc.png">
</div>
<!-- container holds front of all 3 cards visa mc or generic-->
<div class="mycard_front">
<img class="img-fluid center-block as_gen card_size" src="img/gen.png">
<img class="img-fluid center-block as_visa card_size" src="img/visa.png" style="display: none;">
<img class="img-fluid center-block as_mastc card_size" src="img/mc.png" style="display: none;">
</div>
</div>
<!--Place holder block to keep the space available as the absolut position card flips-->
<img class="img-responsive center-block card_size" src="img/gen.png" style="visibility: hidden;">
</div>
CSS
<style>
/* the card css*/ div#mycard { position: relative; margin:20px auto; max-width: 435px; } div.mycard_inner { position:absolute; color:#000; font-size: 17px; font-weight:bold; max-width: 405px; width: 95%; top:18%; line-height:15px; left:10px; } div.card_content { padding:0px 20px 0px 30px; } img.card_size { max-width:434px;width:100%; } p.cardholdername { text-transform: uppercase; } p.cardvalue { font-size: 1.5rem; padding-bottom: 28%; } /* hide cc number when card flips */ div#mycard.hover p.cardvalue { opacity: 0; } /* this fixes visibility issues on safari for OSX*/ #mycard { -webkit-perspective: 1000; -moz-perspective: 1000; -o-perspective: 1000; perspective: 1000; } /*cvv initially hidden on front*/ p.p_cvv { visibility: hidden; margin-top: -30px; } #mycard.hover .flipper { -webkit-transform: rotateY(180deg); transform: rotateY(180deg); } #mycard.hover .flipper { transform: rotateY(180deg); } .flipper { -webkit-transition: 0.6s; transition: 0.6s; -webkit-transform-style: preserve-3d; transform-style: preserve-3d; position: relative; } /* hide back of pane during swap */ /* vendor prefix fixes iOS bug not showing back of card after flip*/ .mycard_front, .mycard_back { -webkit-backface-visibility: hidden; -moz-backface-visibility: hidden; -o-backface-visibility: hidden; -webkit-transition: 0.6s; -webkit-transform-style: preserve-3d; -moz-transition: 0.6s; -moz-transform-style: preserve-3d; -o-transition: 0.6s; -o-transform-style: preserve-3d; -ms-transition: 0.6s; -ms-transform-style: preserve-3d; transition: 0.6s; transform-style: preserve-3d; position: absolute; top: 0; left: 0; } /* front of card, placed above back */ .mycard_front { z-index: 0; -webkit-transform: rotateY(0deg);/* for firefox */ transform: rotateY(0deg); } #mycard.hover .mycard_front { z-index: -10; } /* back of card, initially hidden */ .mycard_back { -webkit-transform: rotateY(180deg);/* for firefox */ transform: rotateY(180deg); } #mycard.hover .mycard_back { -webkit-transform: rotateY(180deg); -moz-transform: rotateY(180deg); -o-transform: rotateY(180deg); -ms-transform: rotateY(180deg); transform: rotateY(180deg); } div.mycard_inner { z-index: 1; } @media (max-width: 1200px) { p.cardvalue { } } @media (max-width: 992px) { div.mycard_inner { font-size: 15px; line-height: 11px; } p.cardvalue { } } @media (max-width: 768px) { #mycard { } label { font-size: 11px; } img.ccImg { max-width: 90px; margin-top: 5px; } /* make cc number smaller on smaller devices */ p.cardvalue { font-size:1.4rem; } } @media (max-width: 420px) { p.cardvalue { font-size:1.1rem; } } @media (max-width: 320px) { p.cardvalue { font-size:.85rem; } }</style>
jQuery/Javascript
<script type="text/javascript">
function formatmycard() { var mycardvalue = $('input[name=ccn]').val(); //lets check for mastercard and visa var mastercard_chk = mycardvalue.match(/^(?:5[1-5][0-9]{2}|222[1-9]|22[3-9][0-9]|2[3-6][0-9]{2}|27[01][0-9]|2720)[0-9]{0,12}/g); var visa_chk = mycardvalue.match(/^4[0-9]{0,12}(?:[0-9]{3})?/g); if (mastercard_chk != null) { console.log(mastercard_chk); console.log('mastercard'); $("img.as_gen,img.as_visa").hide(); $("#mycard img.as_mastc").show(); } else if (visa_chk != null) { console.log(visa_chk); console.log('visa'); $("img.as_gen,img.as_mastc").hide(); $("#mycard img.as_visa").show(); } else { $("img.as_visa,img.as_mastc").hide(); $("#mycard img.as_gen").show(); } //Get/Set - get the card number, format it, then then display it on the card var myformattedcard = mycardvalue.replace(/(\d{4}(?!\s))/g, "$1 "); $('p.cardvalue').text(myformattedcard); } //listen for user to type their card number $("input[name=ccn]").keyup(function(){ formatmycard(); }); // show back of the card when they select cvv $("input[name=cvv]").focus(function(){ $('#mycard').addClass('hover'); $('p.p_cvv').css('visibility','visible'); $('p.cardholdername,p.exp').css('visibility','hidden'); }); //show front of card when they leave the cvv input $("input[name=cvv]").blur(function(){ $('#mycard').removeClass('hover'); $('p.p_cvv').css('visibility','hidden'); $('p.cardholdername,p.exp').css('visibility','visible'); }); // let's display the cvv on the card when they type $("input[name=cvv]").keyup(function(){ var mycvvvalue = $('input[name=cvv]').val(); //regex here if reformatting needed $('small.cvvval').text(mycvvvalue); }); //display the exp month $("select[name=expM]").change(function(){ var myexpmvalue = $('select[name=expM]').val(); $('span.expm').text(myexpmvalue); }); //display the exp year $("select[name=expY]").change(function(){ var myexpyvalue = $('select[name=expY]').val(); $('span.expyr').text(myexpyvalue); });</script>
<script type="text/javascript">
//initialize our formatmycard() function with jQuery Document Ready function $(document).ready(function(){ formatmycard(); });</script>
What's Needed
- jQuery CSS & Javascript
- Include jQuery in the
<head>
- Images of Visa and Mastercard Credit Cards
.match()
should have a clear understanding of this javascript method - match() on MOZ- We can do this with a combination of plain JS & HTML and CSS
- This should be cross browser compatible and work on mobile devices
- Some CSS transitions
The "trick" here is to show the credit card update as the user is typing in the form. This way they know the page is updating the display in realtime and no funky loaders are needed. This is a little more advanced of a script but once you master all the methods involved you can open this up for further customization.
Updated: Jan 2022
Feel free to share this Source Code and use on your personal Website Projects.
All scripts and snippets authored by https://www.andysotura.com