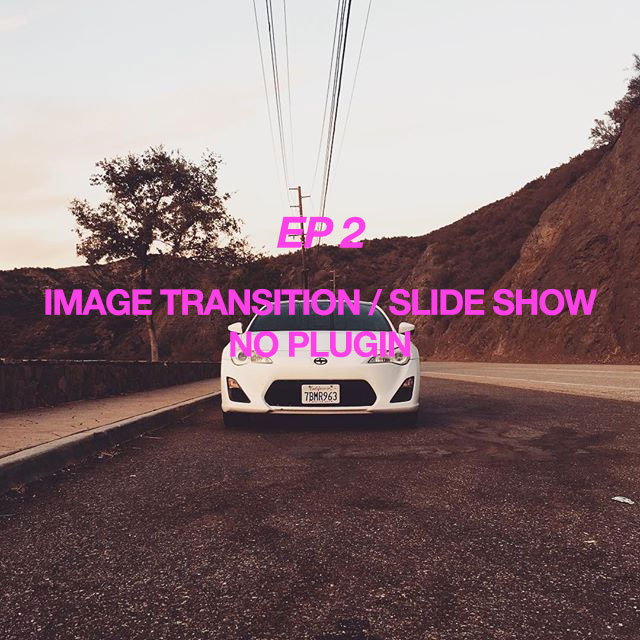
I noticed a lot of developers using plugins for such a simple task....enough owl, bx, nuvo, and flickity sliders...let's see how simple we can make this snippet
OUR PROBLEM
You're asked to create a landing page with several product photos. You're given a few assets but figure hey these will look good in a slideshow. Before you go looking up "best javascript sldeshow plugin" let's see how easy it is to write in it's most basic form.
Why You might need this
- You have a few images that need to be shown in a block
- Your real estate is limited on this landing page so you want to use one block to rotate through several images.
- You want to lower you page footprint by not including any additional libraries or scripts.
ANSWER: Let's Use Javascript
You could spend hours playing with settings on a slideshow plugin OR you could use JavaScript to rotate through each image one by one while saving kb on the additional library you're not loading.
View Code Example Below:
PSEUDO CODE
//Let's define our array of images
//Let's preload our images to the DOM
//** Very important to have these files preloaded before we run our script
//at the end of the preload loop let's run our function (this way we know the files were loaded first)
//Write a function that changes the src of the image every 2 seconds (length of time we want the image to show for)
HTML
<img id="pic_block" src="/img/ep2.jpg" class="img-fluid" style="width:100%;">
Javascript
<script type="text/javascript">
var mypics = ["/img/trek1.jpg","/img/trek2.jpg","/img/trek3.jpg","/img/trek4.jpg"]; var pic_block = document.getElementById("pic_block"); function preload_img(url) { var img=new Image(); img.src=url; } function slideshow(count,length){ var i=0; setInterval(function(){ pic_block.src = mypics[i]; i++; if(i==count)i=0; },length); } onload=function(){ for(var i=0;i<mypics.length;i++){ preload_img(mypics[i]); if (i==mypics.length-1){ slideshow(4,2000); } } }</script>
What's Needed
- several jpg images that you want to rotate on the page
- an HTML img element that can be replaced on page load
- Preload images
setInterval()
should have a clear understanding of this javascript method - setInterval() on MOZ- We can do this with a combination of plain JS & HTML and CSS
- This should be cross browser compatible and work on mobile devices
- Pure Javascript no jQuery is needed on this one...but can easily be redone with jQuery
The "trick" here is to write a simple slideshow with a very small code footprint. We use setInterval
and the .src
method to create a very simple slideshow. In Part 2, we'll revisit this and apply some fancy CSS transitions to make it "swipe" or move.
Updated: Jan 2022
Feel free to share this Source Code and use on your personal Website Projects.
All scripts and snippets authored by https://www.andysotura.com