You ever watch a gif and it just makes you feel some type of way? Well, what if I told you that gifs could be used to create web video experiences that elicit those same types of emotions? Believe it or not, GIFs can be used as a form of web video. In this article, we'll show you how to use GIFs like web videos by using JavaScript.
OUR PROBLEM
Your Boss tells you to create a landing page with the video playing but sends you 4 .gif files each 3 seconds in length. What do you do now?
Why You might need this
- Your video clip is large (more than 10MB) and you're trying to save on overall page size
- You want a video to seem like it's playing above the fold on page load (without html 5 video tag)
- You're spending a lot of money on mobile traffic and want to make sure you save
- You work in the affiliate business and need to make visitors believe they are watching a video on their mobile device without clicking anything (user gestures...)
ANSWER: Let's Use Javascript
You could create a landing page with all of the gifs playing at once, but that would be overwhelming for the viewer. OR you could use JavaScript to play each gif one by one and create a more seamless experience for your viewers.
View Code Example Below:
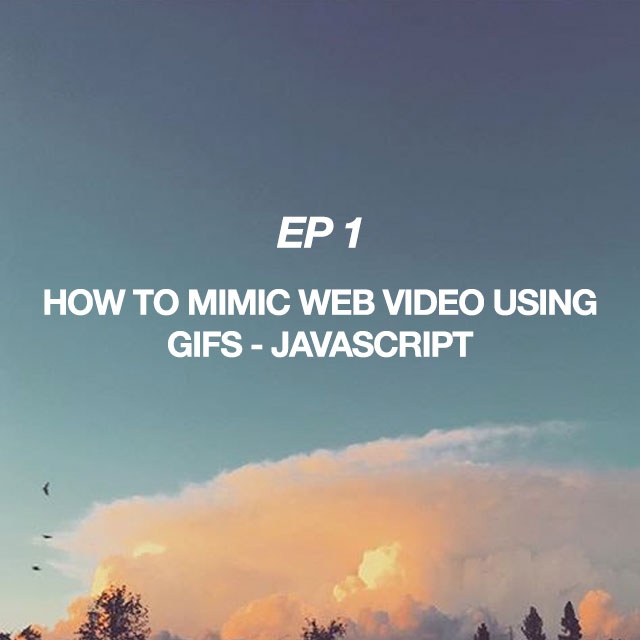
PSEUDO CODE
//Let's define our array of GIF images
//Let's preload our images to the DOM
//** Very important to have these files preloaded before we run our script
//at the end of the loop let's run our function
//Write a function that accepts an image and loads it on the page ever 3 seconds (length of gif file)
HTML
<img id="gifblock" src="/img/ep1.jpg" class="img-fluid" style="width:100%;">
Javascript
<script type="text/javascript">
var mygifs = ["/img/gifs/1n.gif","/img/gifs/2n.gif","/img/gifs/3n.gif","/img/gifs/4n.gif"]; var gifholder = document.getElementById("gifblock"); function preload_img(url) { var img=new Image(); img.src=url; } function playgif(count,length){ var i=0; setInterval(function(){ gifholder.src = mygifs[i]; i++; if(i==count)i=0; },length); } onload=function(){ for(var i=0;i<mygifs.length;i++){ preload_img(mygifs[i]); if (i==mygifs.length-1){ playgif(4,3000); } } }</script>
What's Needed
- 1 video clip split up into equal durations .gif files (each GIF is 3 seconds)
- GIF duration tool - I use this tool for even out the gifs duration
- an HTML img element that can be replaced on page load
- Preload images is a must do here - When visitors see the page for the first time they dont need to wait for each gif file to load since the images are already preloaded
setInterval()
should have a clear understanding of this javascript method - setInterval() on MOZ- Use when your page has lots of gifs to mimic web video being played without user interaction
- We can do this with a combination of plain JS & HTML
- This should be cross browser compatible and work on mobile devices
- Pure Javascript no jQuery is needed on this one...but can easily be redone with jQuery
The "trick" here is to string all the gifs together with javascript making it appear as if a video is playing. For anyone with paid traffic this is a must. We don't want to tarnish the visitor experience waiting for a GIF to load with would appear as a broken page. Loading an entire video is a gamble since you have no way of knowing the speed of your users connection.
Updated: Jan 2022
Feel free to share this Source Code and use on your personal Website Projects.
All scripts and snippets authored by https://www.andysotura.com